In Salesforce Lightning Component, <aura:method> is powerful and is supported for code reuse. The use of <aura:method> simplifies the code needed for a parent component to call a method on a child component that it contains. The <aura:method> enables you to directly call a method in a component’s client-side controller instead of firing and handling a component event.
<aura:component>
<aura:method name="myMethod" action="{!c.myAction}"
description="This a Sample method in a child component " access= global>
</aura:method>
</aura:component>
The following are the system attributes of <aura:method> tag :
- Name – The method name
- Action – Use by the client-side controller
- Access – This can be public or global
- Description – The method description
If the action value is not specified, the controller action defaults to the value of the method name.
To add parameters to the <aura:method> tag, use <aura:attribute> tag within it.
<aura:component>
<aura:method name="myMessage"
description="Sample method with parameters">
<aura:attribute name="name" type="String" default="parameter 1"/>
<aura:attribute name="message" type="String" default="parameter 2"/>
</aura:method>
</aura:component>
The aura:method above has two parameters – name and message. These attributes enable you to set name and message parameters when you call the myMessage method.
The name attribute of myMessage configures the aura:method to invoke myMessage() in the client-side controller.
Implement the aura:method in child Component and controller
Child Component
<aura:component >
<aura:method name="myMessage" action="{!c.callMessage}"
description="Sample method with two parameters">
<aura:attribute name="title" type="String" default="param 1"/>
<aura:attribute name="name" type="String" default="param 2"/>
<aura:attribute name="message" type="String" default="parameter 3"/>
</aura:method>
</aura:component>
Child Controller – aMethodChildController.js
callMessage : function(component, event) {
var params = event.getParam("arguments");
if (params) {
var title = params.title;
var name = params.name;
var message = params.message;
console.log("Title: "+ title +", "+"Name: " + name +", " + "message: " + message);
let retParams = title + name + message;
return retParams;
}
}
The callMessage in the code correspond to the action value and the three parameters in the component are used in the controller. Be careful with how you represent the parameters because Javascript is case-sensitive
Note: In the aura:method markup, you don’t explicitly declare a return value. Just use the statement in the JavaScript controller. In this child controller, if you comment out the return setParams and implement the aMethodParentController.js, the aura method result of amResult will be undefined.
Implement aura:method in Parent Component and Controller
<!--aMethodParent.cmp -->
<aura:component implements="force:appHostable,flexipage:availableForAllPageTypes" access="global">
<p>Parent component calls aura method in child component</p>
<c:aMethodChild aura:id="amChild"/> //assign id to the child component
<lightning:button label="Call Child Component"
onclick="{!c.callChildAMethod}"/>
</aura:component>
Parent Controller – aMethodParentController.js
callChildAMethod : function(component, event, helper) {
var childCmpt = component.find("amChild"); //the id is used here
//call the aura:method by the name value in the child component
var amResult = childCmpt.myMessage("Chief ", "Lola ", "send this parent component message");
console.log("Aura Method Result: " + amResult);
}
The childCmpt.myMessage(“Chief “, “Lola “, “send this parent component message”); follows the Syntax – cmp.myMethod(arg1, … argN); for calling a method in JavaScript code where
cmp is a reference to the component.
myMethod is the name of the aura:method,
arg1, … argN is an optional comma-separated list of arguments passed to the method. Each argument corresponds to an aura:attribute defined in the aura:method markup. In this example, the three parameters are the title, name and message while the arguments parsed are Chief, Lola and the message.
The result of the above code after clicking the callChildMethod button is pasted below :
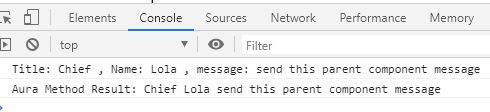
Reference