There are some use cases where you need to display List – an aura:attribute collection type as picklist in Lightning Component to display array of items.
A list is an ordered collection of items. It is one of the <aura:attribute> supported collection type values.
To set items in a list using a client-side controller, create an attribute of type List and set the items using component.set().
The example below – ListCom.cmp retrieves a list of even numbers from a client-side controller when a button is clicked.
<aura:component >
<aura:attribute name="numbers" type="List"/>
<lightning:button onclick="{!c.getEvenNumbers}" label="Display Even Numbers" />
<aura:iteration var="num" items="{!v.numbers}">
<div>{!num.value}</div>
</aura:iteration>
</aura:component>
Consider the ListCompController.js
({
getEvenNumbers: function(component, event, helper) {
var numbers = [];
for (var i = 0; i < 20; i++) {
if(i % 2 == 0){
numbers.push({
value: i
});
}
component.set("v.numbers", numbers);
}
}
})
Create a Lightning Application and add <c:ListCmp /> to it. The result you get when you click the Display Even Numbers button on the page is attached below
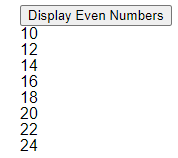
Display the List as Picklist
To display these array as picklist, the code for both the markup and the client-server side controller are added below.
For the markup – ListPicklistComp.cmp
<aura:component >
<aura:attribute name="numbers" type="List"/>
<!--lightning:button onclick="{!c.getEvenNumbers}" label="Display Even Numbers" / There is no need for a button-->
<aura:handler name="init" value="{!this}" action="{!c.getEvenNumbers}"/>
<lightning:select aura:id="evenNum" name="evenNum" label="Even Numbers">
<aura:iteration items="{!v.numbers}" var="nums">
<option value="{!nums}">{!nums}</option>
</aura:iteration>
</lightning:select>
</aura:component>
For the ListPicklistCompController.js
({
getEvenNumbers: function(component, event, helper) {
var numbers = [];
for (var i = 10; i < 25; i++) {
if(i % 2 == 0){
numbers.push(i);
}
}
component.set("v.numbers", numbers);
}
})
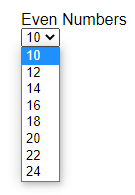
The difference between the ListPicklistCompController.js code and the other ListCompController.js is the array push method. Using the later controller code results in getting [object Object] for the values instead of the expected values as shown in the Even Numbers dropdown above.
To create a form with multiple picklist fields in Lightning Component, you can check my post on the subject – Create a form with Picklist fields in Lightning Component
If there is any question on this post or any other one in my blog, feel free to contact me. I will be glad to help.